Flutter: Integrating Socket IO Client
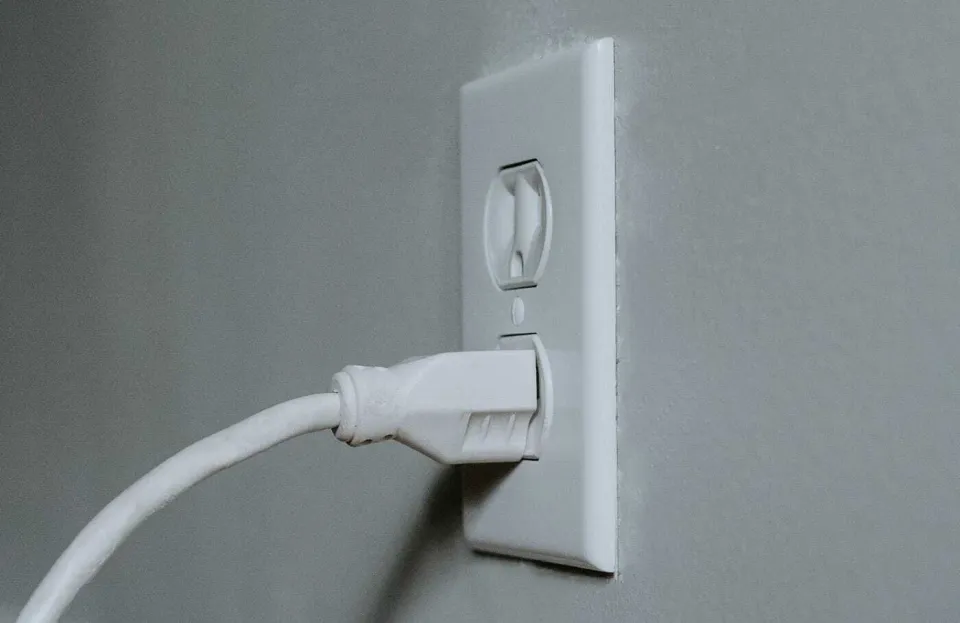
What is Web Socket & Socket IO?
Web Sockets: Web Sockets is a communication protocol that provides full-duplex communication channels over a single TCP connection. It enables real-time, bidirectional communication between a client (typically a web browser) and a server. Unlike traditional HTTP requests, where the client sends a request and the server responds, Web Sockets allow for continuous, ongoing communication between the client and the server.
Web Sockets provide a persistent connection that remains open, allowing both the client and server to send data to each other at any time without the need for frequent HTTP request-response cycles. This real-time communication capability is especially useful for applications that require instant updates or interactive features, such as chat applications, real-time gaming, collaborative editing, and live data streaming.
Web Sockets use a simple messaging protocol and are supported by most modern web browsers and web servers. They provide a standardized way for web applications to establish and maintain a long-lived connection for efficient, low-latency, and real-time communication.
Socket.IO: Socket.IO is a JavaScript library that provides real-time, event-based communication between web clients and servers. It is built on top of Web Sockets but also supports alternative transport mechanisms, such as long polling, if Web Sockets are not available. Socket.IO abstracts away the underlying transport layer and provides a consistent API for real-time communication across different browsers and platforms.
Socket.IO simplifies the process of implementing real-time functionality in web applications. It provides features like automatic reconnection, event-based messaging, and support for broadcasting messages to multiple clients. Socket.IO also supports namespaces and rooms, allowing you to organize clients into different groups for targeted communication.
Socket.IO is widely used in web development for building real-time applications, including chat applications, collaborative tools, real-time analytics, and live dashboards. It has client libraries available for various platforms, including JavaScript for web browsers, as well as libraries for popular server-side technologies such as Node.js, Python, and Java.
By leveraging Web Sockets and fallback mechanisms, Socket.IO ensures that real-time communication can be established even in environments where direct Web Socket connections may not be possible, providing a robust and versatile solution for real-time web applications.
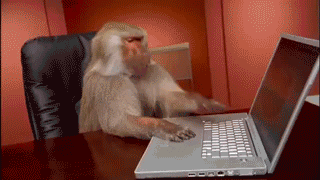
How to Code?
1.Import the package: First, you need to include the socket_io_client package in your Flutter project. You can add it to your pubspec.yaml
file and run flutter pub get
to fetch the package.
2.Create a SocketIO instance: To establish a connection to the Socket.IO server, you create an instance of SocketIO
class. The constructor takes the server URL as a parameter.
import 'package:socket_io_client/socket_io_client.dart' as io;
final socket = io.io('http://example.com');
3.Configure the connection: You can configure the connection options using OptionBuilder
methods. For example, you can set the transports (e.g., WebSocket) to be used, disable auto-connection, add extra headers, and more.
Here’s an example:
import 'package:socket_io_client/socket_io_client.dart' as io;
final socket = io.io(
'http://example.com',
io.OptionBuilder()
.setTransports(['websocket'])
.disableAutoConnect()
.setExtraHeaders({'x-auth-token': 'your-auth-token'})
.enableForceNew()
.build(),
);
4.Handle events: Socket.IO is event-driven, so you can define event listeners to handle incoming events from the server. You can use the on
method to listen to specific events.
For example:
socket.on('getMessage', (newMessage) {
messageList.add(MessageModel.fromJson(data));
});
5. Emit events: You can also send events from the client to the server using the emit
method. Specify the event name and the data to be sent.
For example:
sendMessage() {
String message = textEditingController.text.trim();
if (message.isEmpty) return;
Map messageMap = {
'message': message,
'sender_id': userId,
'receiver_id': receiverId,
'message_type': messageType,
};
socket.emit('sendNewMessage', messageMap);
}
6. Connect and disconnect: To establish a connection to the server, you can call the connect
method on the socket object. Likewise, to disconnect from the server, you can use the disconnect
method
socket.connect();
// ...
socket.disconnect();
7. Error handling: Socket.IO provides error handling mechanisms. You can listen for error events and handle them appropriately.
For example:
socket.on('connect_error', (error) {
print('Connection error: $error');
});
These are the basic steps to use Socket.IO on the client side in Flutter. You can explore the documentation of the socket_io_client package for more details on additional features and options available for Socket.IO in Flutter.