Reducing Cloud Costs for Your Development Environment with AWS Lambda and CloudWatch
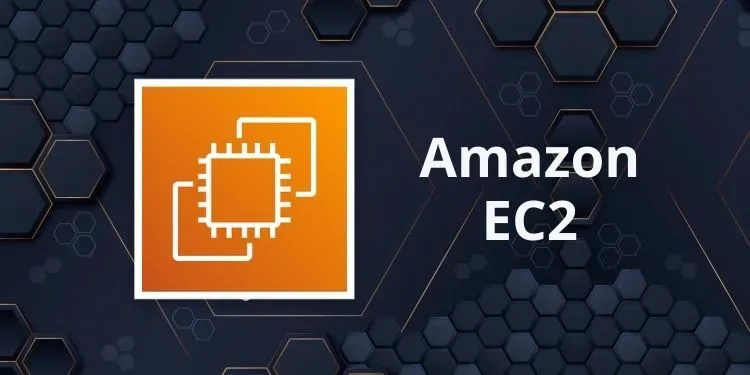
For businesses and developers, the cost of cloud computing can quickly add up, especially for development environments. However, by automating processes and utilizing tools like AWS Lambda and CloudWatch, it’s possible to reduce cloud costs and make your development environment more efficient. In this article, we’ll show you how to set up an EC2 instance, start a service with PM2, create a Lambda function to start and stop the EC2 instance, and finally, set up CloudWatch events to trigger the Lambda function.
Setting up EC2 Instance with Elastic IP on AWS
Step 1: Log into your AWS account
To start the process, you will need to log into your AWS account. You can do this by visiting the AWS website and entering your credentials.
Step 2: Launch the EC2 instance
Once you are logged in, go to the EC2 dashboard and click on ‘Launch Instance.’ You will see a list of available Amazon Machine Images (AMIs). Select the AMI that you want to use for your instance and click ‘Select.’
Step 3: Choose an instance type
Now, you need to choose an instance type that fits your requirements. AWS provides a variety of instance types, ranging from small to large instances. Based on your needs, choose an instance type and click ‘Next.’
Step 4: Configure instance details
In this step, you will configure the instance details. You will have to specify the number of instances you want to launch and the network settings. You can also choose the subnet and security group that you want to use. After you have made all the required configurations, click ‘Next.’
Step 5: Add storage
In this step, you will add storage to your instance. You can add an Amazon Elastic Block Store (EBS) volume to your instance, or you can use an instance store. Choose the storage option that you want to use and click ‘Next.’
Step 6: Add tags
You can add tags to your instance to identify it. This is an optional step, but it is a good practice to add tags to your instances. You can add tags such as ‘Name,’ ‘Environment,’ etc. After adding the tags, click ‘Next.’
Step 7: Configure security groups
In this step, you will configure the security groups for your instance. Security groups control the inbound and outbound traffic to your instance. You can create a new security group or use an existing one. After you have made the required configurations click ‘Review and Launch.’
Step 8: Launch the Instance
In this step, you will launch the instance. You will have to select the key pair that you want to use to connect to the instance. If you do not have a key pair, you can create one. After you have selected the key pair, click ‘Launch Instances.’
Step 9: Assign an Elastic IP
After launching the instance, you will need to assign an Elastic IP to it. To do this, go to the EC2 dashboard and click on ‘Elastic IPs.’ Click on ‘Allocate new address’ and assign the Elastic IP to your instance.
Step 10: Connect to the instance
Finally, you will need to connect to the instance. You can do this using an SSH client such as PuTTY. Enter the Elastic IP of your instance, and you will be able to connect to it.
Setting up the project and starting the service via PM2 on AWS
Step 1: Connect to the EC2 instance
To start, you need to connect to the EC2 instance that we set up in the previous part. You can do this using an SSH client such as PuTTY. Enter the Elastic IP of your instance, and you will be able to connect to it.
Step 2: Install Node.js and npm
The next step is to install Node.js and npm on the EC2 instance. To do this, run the following command in the terminal:
sudo apt-get update
sudo apt-get install nodejs
sudo apt-get install npm
Step 3: Install PM2
After installing Node.js and npm, you need to install PM2. To do this, run the following command:
sudo npm install pm2 -g
Step 4: Clone the project
Now, you need to clone the project that you want to run on the EC2 instance. You can do this using Git. Run the following command:
git clone <project-repository>
Step 5: Change to the project directory
After cloning the project, you need to change to the project directory. Run the following command:
cd <project-directory>
Step 6: Install dependencies
Before starting the service, you need to install the dependencies required by the project. You can do this by running the following command:
npm install
Step 7: Start the service
Now, you are ready to start the service. You can do this by running the following command:
pm2 start <service-file>
Step 8: Save the PM2 process list
After starting the service, you need to save the PM2 process list. This is important because if the EC2 instance restarts, the PM2 process list will be lost, and your service will stop. To save the PM2 process list, run the following command:
pm2 save
Step 9: Add PM2 startup
The last step is to add a PM2 startup. This means that PM2 will start automatically when the EC2 instance restarts. To add PM2 startup, run the following command:
pm2 startup
Creating a Lambda function to start and stop the EC2 instance using JavaScript and the AWS SDK
Step 1: Log in to the AWS Console
To start, you need to log in to the AWS Console. You can do this by navigating to the AWS website and logging in with your credentials.
Step 2: Create a new Lambda function
Once you are logged in, you need to create a new Lambda function. To do this, navigate to the Lambda section in the AWS Console and click on the “Create function” button.
Step 3: Select the runtime environment
When creating a new Lambda function, you need to select the runtime environment. In this case, we will be using JavaScript. So, select Node.js as the runtime environment.
Step 4: Create a new role
Next, you need to create a new role for the Lambda function. To do this, click on the “Create a new role from one or more templates” option and select the “Simple Microservice permissions” template.
Step 5: Add the AWS SDK
The next step is to add the AWS SDK to the Lambda function. This will allow you to interact with the EC2 instance from the Lambda function. To do this, you need to add the following code to the function:
const AWS = require("aws-sdk");
AWS.config.update({
accessKeyId: <YOUR_ACCESS_KEY>,
secretAccessKey: <YOUR_SECRET_ACCESS_KEY>,
region: <YOUR_REGION_EC2>
});
const ec2 = new AWS.EC2();
Step 6: Write the start and stop functions
After adding the AWS SDK, you need to write the start and stop functions for the EC2 instance. You can do this by adding the following code to the function:
exports.handler = async (event) => {
switch (event.operation) {
case "start":
return startInstance(event.instanceId);
case "stop":
return stopInstance(event.instanceId);
default:
throw new Error(`Unsupported operation "${event.operation}"`);
}
};
async function startInstance(instanceId) {
const params = {
InstanceIds: [instanceId],
};
try {
await ec2.startInstances(params).promise();
return { message: `Successfully started instance ${instanceId}` };
} catch (error) {
return { error: `Failed to start instance ${instanceId}: ${error.message}` };
}
}
async function stopInstance(instanceId) {
const params = {
InstanceIds: [instanceId],
};
try {
await ec2.stopInstances(params).promise();
return { message: `Successfully stopped instance ${instanceId}` };
} catch (error) {
return { error: `Failed to stop instance ${instanceId}: ${error.message}` };
}
}
Step 7: Add the IAM role to the EC2 instance
Finally, you need to add the IAM role to the EC2 instance. This will allow the Lambda function to interact with the EC2 instance. To do this, navigate to.
Setting up CloudWatch events to trigger the Lambda function
Step 1: Log in to the AWS Console
To start, you need to log in to the AWS Console. You can do this by navigating to the AWS website and logging in with your credentials.
Step 2: Navigate to the CloudWatch section
Once you are logged in, you need to navigate to the CloudWatch section in the AWS Console.
Step 3: Create a new rule
Next, you need to create a new rule. To do this, click on the “Create rule” button.
Step 4: Select the trigger
When creating a new rule, you need to select the trigger. In this case, we will be using the “Cron” trigger. So, select the “Cron” option.
Step 5: Define the schedule
Next, you need to define the schedule for the CloudWatch event. You can do this by specifying the cron expression. For example, you can use the following expression to trigger the event every day at 8 PM:
0 20 * * *
Step 6: Select the target
After defining the schedule, you need to select the target for the CloudWatch event. In this case, you need to select the Lambda function that you created in part 3.
Step 7: Repeat the process for the stop event
Finally, you need to repeat the above steps to create a second CloudWatch event that will trigger the Lambda function to stop the EC2 instance. You can use a different cron expression to trigger the stop event, for example:
0 8 * * *
Conclusion
By implementing the steps outlined in this article, you can save money and reduce your cloud costs for your development environment. The use of AWS Lambda and CloudWatch events will help you automate processes, reducing manual work and increasing efficiency, making it easier to focus on other important tasks. Whether you’re a business or a developer, using these tools will give you more control over your cloud environment and help you save on cloud costs.